Moe's Tech Blog
[Three.js] About Materials 본문
MeshBasicMaterial
- Creates a simple material object
- No shadow, no textures, just plain solid color
MeshNormalMaterial
- Displays a nice blue, purple and greenish color
- This is because of normal
- Normal is a vector that contains direction outside of the surface
- FlatShading flattens the faces
- MeshNormalMaterial is useful to debug the normals, but it also looks great
import * as THREE from 'three'
const material = new THREE.MeshNormalMaterial();
material.flatShading = true;
MeshMatcapMaterial
- MeshMatcapMaterial is a fantastic material because of how great it looks while being performance-driven
- MeshMatcapMaterial needs a reference texture
- MeshMatcapMaterial picks colors on the texture according to orientation of camera
- Some matcaps can be obtained here
import * as THREE from 'three'
const textureLoader = new THREE.TextureLoader();
const matcapTexture = textureLoader.load("<matcap_image_path>");
const material = new THREE.MeshMatcapMaterial();
material.matcap = matcapTexture;
MeshDepthMaterial
- MeshDepthMaterial colors the geometry in
- 1. White if its close to
near
value of the camera - 2. Black if its close to
far
value of the camera
- 1. White if its close to
- MeshDepthMaterial requires light
import * as THREE from 'three'
const material = new THREE.MeshDepthMaterial();
const pointLight = new THREE.PointLight("#FFFFFF", 0.9);
pointLight.position.x = 2;
pointLight.position.y = 3;
pointLight.position.z = 4;
scene.add(pointLight);
MeshLambertMaterial
- MeshDepthMaterial is a simple material that reacts to light
import * as THREE from 'three';
const material = new THREE.MeshLambertMaterial();
const pointLight = new THREE.PointLight("#FFFFFF", 0.9);
pointLight.position.x = 2;
pointLight.position.y = 3;
pointLight.position.z = 4;
scene.add(pointLight);
MeshPhongMaterial
- MeshPhongMaterial also reacts to light
- MeshPhongMaterial provides reflection off the surface
- Can control shininess through shininess property
- Can control color of light off the surface through specular property
import * as THREE from 'three';
const material = new THREE.MeshPhongMaterial();
material.shininess = 35;
material.specular = new THREE.Color("#FFFFFF");
const pointLight = new THREE.PointLight("#FFFFFF", 0.9);
pointLight.position.x = 2;
pointLight.position.y = 3;
pointLight.position.z = 4;
scene.add(pointLight);
MeshToonMaterial
- MeshMoonMaterial is similar to MeshLambertMaterial but it’s more cartoonish in style
- Gradient textures can be added to show different types of gradients
import * as THREE from 'three';
const gradientTexture = textureLoader.load("/textures/gradients/5.jpg");
gradientTexture.minFilter = THREE.NearestFilter
gradientTexture.magFilter = THREE.NearestFilter
gradientTexture.generateMipmaps = false
const material = new THREE.MeshToonMaterial();
MeshStandardMaterial
- MeshStandardMaterial is like MeshLambertMaterial and MeshPhongMaterial but with more realistic algorithm and better parameters like roughness
- MeshStandardMaterial is called standard because it’s becoming standard in many libraries
import * as THREE from 'three';
const material = new THREE.MeshStandardMaterial();
- Additional properties can be added to add sophistication to object
- 1. aoMap property adds shadow where texture is dark
- aoMap means ambient occlusion map
- For this to work, a second set of uv (coordinate that helps to position textures on geometries) must be added
import * as THREE from 'three';
const doorColorTexture = textureLoader.load("/textures/door/color.jpg");
const doorAmbientOcclusionTexture = textureLoader.load("/textures/door/ambientOcclusion.jpg");
const material = new THREE.MeshStandardMaterial();
material.metalness = 0.45;
material.roughness = 0.65;
material.map = doorColorTexture;
material.aoMap = doorAmbientOcclusionTexture;
material.aoMapIntensity = 1;
...
sphere.geometry.setAttribute('uv2', new THREE.BufferAttribute(sphere.geometry.attributes.uv.array, 2));
plane.geometry.setAttribute('uv2', new THREE.BufferAttribute(plane.geometry.attributes.uv.array, 2));
torus.geometry.setAttribute('uv2', new THREE.BufferAttribute(torus.geometry.attributes.uv.array, 2));
- 2. displacementScale attribute is used to make depth between crevacies truer
- The reason why it looks weird at first is because it lacks verticies and it's too strong
- Solved by adding more verticies and decreasing intensity
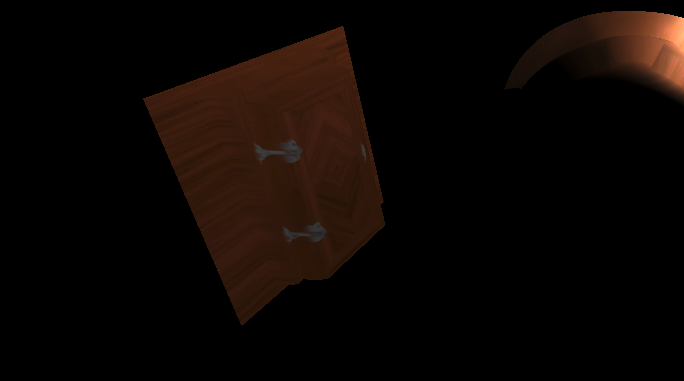
import * as THREE from 'three';
const doorHeightTexture = textureLoader.load("/portfolio-website-v3/textures/door/height.jpg");
const material = new THREE.MeshStandardMaterial();
...
material.displacementMap = doorHeightTexture;
material.displacementScale = 0.03;
const sphere = new THREE.Mesh(
new THREE.SphereGeometry(0.5, 64, 64), // <- used to be 0.5, 16, 16
material
)
const plane = new THREE.Mesh(
new THREE.PlaneGeometry(1, 1, 100, 100), //<- used to be 1, 1
material
)
plane.position.x = -1.5;
const torus = new THREE.Mesh(
new THREE.TorusGeometry(0.3, 0.2, 64, 128), //<- used to be 0.3, 0.2, 16, 32
material
)
- 3. roughnessMap and metalnessMap attributes does roughness and metalness for you
- For this to work, roughness and metalness attributes must be commented out, or set to default values
import * as THREE from 'three';
const doorMetalnessTexture = textureLoader.load("/portfolio-website-v3/textures/door/metalness.jpg");
const doorRoughnessTexture = textureLoader.load("/portfolio-website-v3/textures/door/roughness.jpg");
const material = new THREE.MeshStandardMaterial();
material.metalnessMap = doorMetalnessTexture;
material.roughnessMap = doorRoughnessTexture;
material.metalness = 0;
material.roughness = 1;
- 4. normalMap adds fake normal information and refines detailed depth in geometry
- can be adjusted using normalScale attribute
import * as THREE from 'three';
const doorNormalTexture = textureLoader.load("/textures/door/normal.jpg");
const material = new THREE.MeshStandardMaterial();
material.normalMap = doorNormalTexture;
material.normalScale.set(0.5, 0.5);
- 5. alphaMap adds transparency
import * as THREE from 'three';
const doorNormalTexture = textureLoader.load("/textures/door/normal.jpg");
const material = new THREE.MeshStandardMaterial();
material.transparent = true;
material.alphaMap = doorAlphaTexture;
MeshPhysicalMaterial
- MeshPhysicalMaterial is the same as MeshStandardMaterial but with support for clear coating
- Don't use it unless necessary. It requires more GPU
PointsMaterial
- Creates geometry of material using particles.
- See example here
ShaderMaterial and RawShaderMaterial
- ShaderMaterial and RawShaderMaterial are used to create userdefined, custom materials
Environment map
- Is used to add reflections of the surrounding scene.
Where to find environment maps
Poly Haven • Poly Haven
The Public 3D Asset Library
polyhaven.com
- HDRI must be converted to cube map before use. The tool is found here
Source
- 1. Three.js journey, Bruno Simon : https://threejs-journey.com/
'Three.js > Notes' 카테고리의 다른 글
[Three.js] Creating a Scene in Blender (0) | 2022.05.02 |
---|